This notebook gives the basic steps in order to perform an azimuthal integration, including pixel splitting, of a scattering pattern recorded with the DSSC detector which has hexagonal spahed pixels.
[1]:
%matplotlib inline
import numpy as np
import matplotlib.pyplot as plt
plt.rcParams['figure.constrained_layout.use'] = True
DSSC geometry¶
From the quadrants position and the position of the modules inside each quadrants we compute a DSSC geometry
[2]:
from extra_data import open_run
from extra_data.components import DSSC1M
from extra_geom import DSSC_1MGeometry
quad_pos = [(-122.21101449000001, 2.17217391),
(-124.4, -118.94881159),
(1.32336232, -120.39306931),
(3.1934492800000003, 0.80901765)]
geom_file = "/gpfs/exfel/exp/SCS/201901/p002212/usr/Shared/Training_UP-2719/geometry/dssc_geom_AS_aug20.h5"
geom = DSSC_1MGeometry.from_h5_file_and_quad_positions(geom_file, quad_pos)
We then give this information to pyFAI by creating a detector object from the individuals 6 corner position of each one million pixels.
[3]:
from pyFAI.azimuthalIntegrator import AzimuthalIntegrator
ai = AzimuthalIntegrator(detector=geom.to_pyfai_detector())
WARNING:silx.opencl.common:Unable to import pyOpenCl. Please install it from: https://pypi.org/project/pyopencl
Load the test data¶
We then load some test data to test the azimuthal integration.
[4]:
import xarray as xr
path = '/gpfs/exfel/exp/SCS/202022/p002711/usr/processed_runs/r97'
data = xr.open_mfdataset(path + '/*.h5', parallel=True, join='inner')
data
[4]:
<xarray.Dataset> Dimensions: (pp: 1, bin_nrj: 1, module: 16, y: 128, x: 512, sa1_pId: 200, pulse_slot: 2700) Coordinates: * bin_nrj (bin_nrj) float64 780.0 * module (module) int64 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 * pp (pp) object 'unpumped' * sa1_pId (sa1_pId) int64 600 604 608 612 ... 1384 1388 1392 1396 Dimensions without coordinates: y, x, pulse_slot Data variables: DSSC (pp, bin_nrj, module, y, x) float64 dask.array<chunksize=(1, 1, 1, 128, 512), meta=np.ndarray> SCS_SA1 (module, bin_nrj, sa1_pId) float64 dask.array<chunksize=(1, 1, 200), meta=np.ndarray> SCS_SA3 (module, pp, bin_nrj) float64 dask.array<chunksize=(1, 1, 1), meta=np.ndarray> bunchPatternTable (module, bin_nrj, pulse_slot) float64 dask.array<chunksize=(1, 1, 2700), meta=np.ndarray> nrj (module, bin_nrj) float64 dask.array<chunksize=(1, 1), meta=np.ndarray>
[5]:
img = data['DSSC'].squeeze()
img.shape
[5]:
(16, 128, 512)
[6]:
from matplotlib.colors import LogNorm
from matplotlib.patches import Circle
geom2 = geom.offset((0, 0))
ax = geom2.plot_data_fast(img, colorbar=True, norm=LogNorm(), vmin=1e-3, axis_units='m')
ax.add_patch(Circle((0, 0), radius=0.03, fill=False, color='white'))
[6]:
<matplotlib.patches.Circle at 0x2ba9de743190>
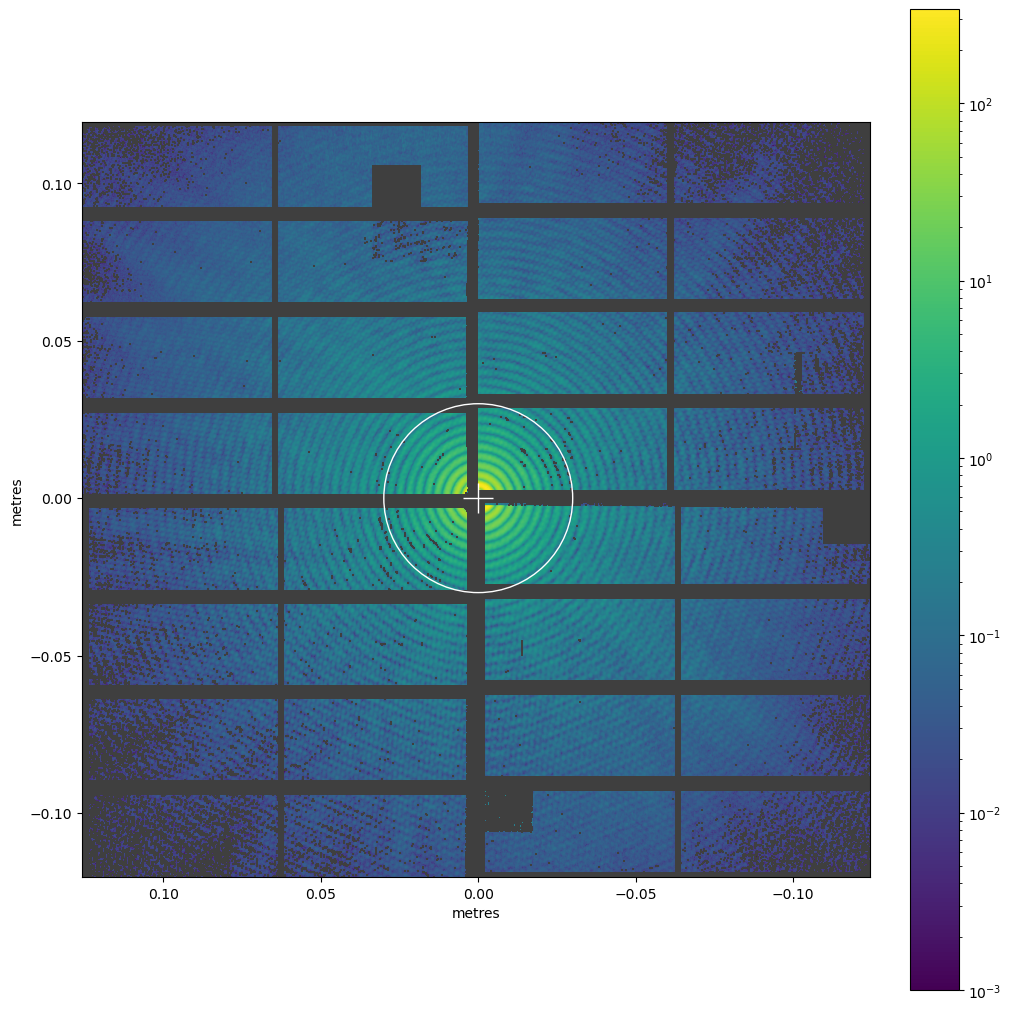
[7]:
joined = img.values.reshape(16*128, 512)
[8]:
cake_bbox, q, chi = ai.integrate2d(
joined,
npt_rad=900,
unit='r_mm',
correctSolidAngle=False,
method="bbox",
)
cake_nosplitting, q, chi = ai.integrate2d(
joined,
npt_rad=900,
unit='r_mm',
correctSolidAngle=False,
method="no",
)
fig, (ax0, ax1) = plt.subplots(ncols=2, figsize=(15, 10))
im0 = ax0.imshow(cake_nosplitting, aspect='auto', norm=LogNorm(), vmin=1e-3)
ax0.set_title('No splitting')
cbar = fig.colorbar(im0, ax=ax0)
im1 = ax1.imshow(cake_bbox, aspect='auto', norm=LogNorm(), vmin=1e-3)
ax1.set_title('bbox splitting')
cbar = fig.colorbar(im1, ax=ax1)
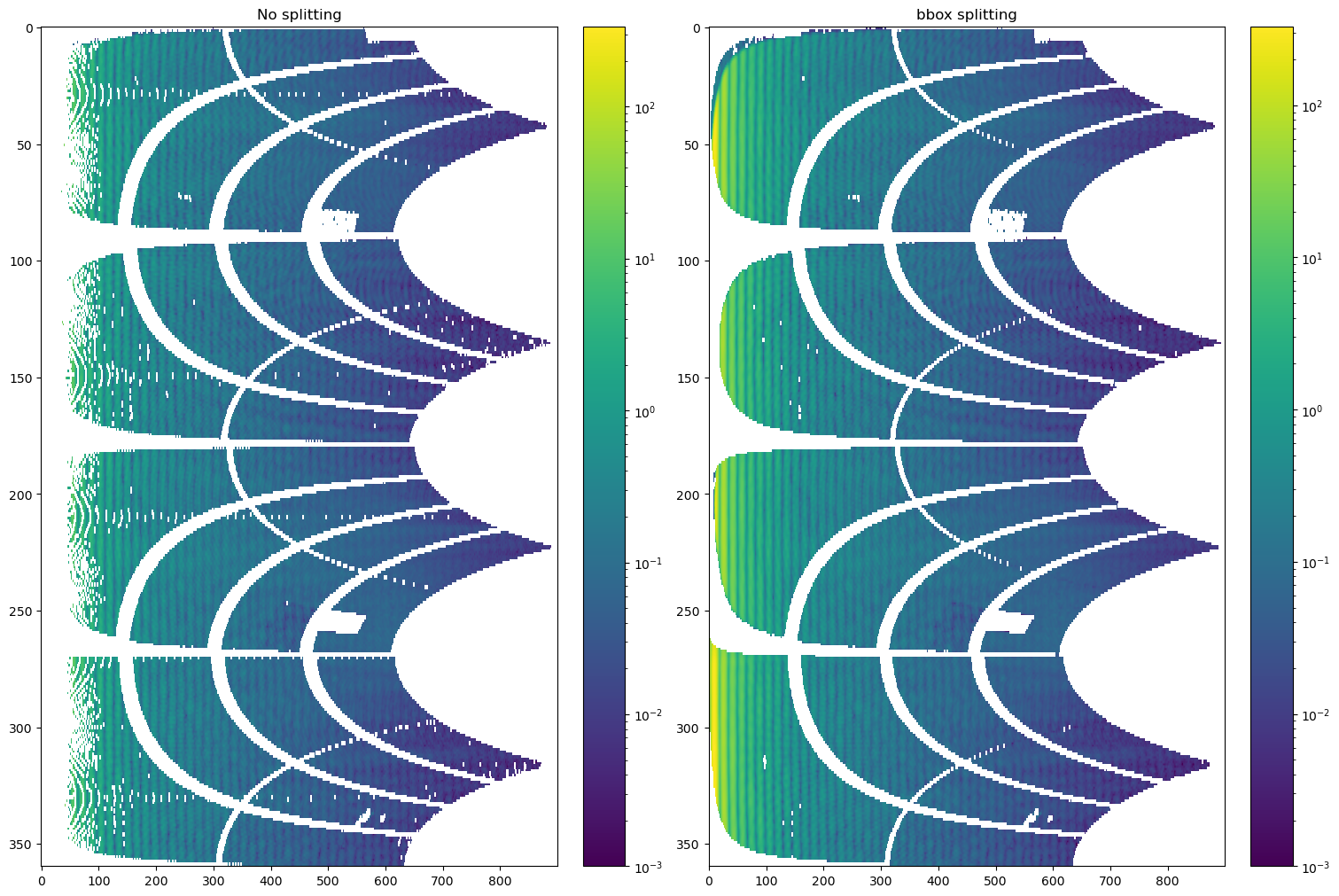
We can confirm that pixel splitting improves the data representation in the low-q range.
[9]:
rint_no, I_no = ai.integrate1d(
joined,
npt=900,
unit='r_mm',
method='no'
)
rint_bbox, I_bbox = ai.integrate1d(
joined,
npt=900,
unit='r_mm',
method='bbox'
)
plt.figure()
plt.semilogy(rint_no, I_no, label='no splitting')
plt.semilogy(rint_bbox, I_bbox, label='bbox splitting')
plt.ylim(bottom=1e-3)
plt.legend()
[9]:
<matplotlib.legend.Legend at 0x2babe9cdf4c0>
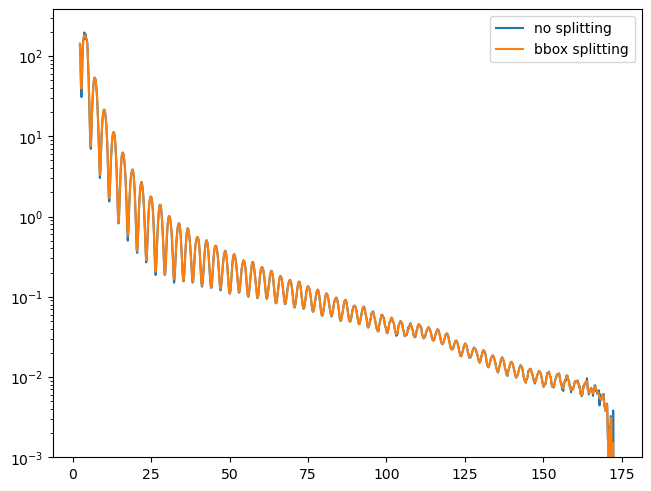
[ ]: