[1]:
import numpy as np
%matplotlib inline
import matplotlib.pyplot as plt
plt.rcParams['figure.constrained_layout.use'] = True
import dask
print(f'dask: {dask.__version__}')
import dask.array
dask.config.set({'array.chunk-size': '512MiB'})
from psutil import virtual_memory
mem = virtual_memory()
print(f'Physical memory: {mem.total/1024/1024/1024:.0f} Gb') # total physical memory available
import logging
logging.basicConfig(filename='example.log', level=logging.DEBUG)
import toolbox_scs as tb
print(tb.__file__)
import toolbox_scs.routines.boz as boz
import os
dask: 2023.3.2
Physical memory: 754 Gb
/home/lleguy/notebooks/ToolBox-git/src/toolbox_scs/__init__.py
[2]:
import sys
print(sys.executable)
/gpfs/exfel/u/scratch/SCS/202301/p003423/envs/toolbox_p003423-gpu/bin/python
[3]:
%load_ext autoreload
%autoreload 2
Create parameters object¶
[4]:
proposal = 2937
darkrun = 615
run = 614
module = 15
gain = 3
drop_intra_darks = True
sat_level = 490
rois_th = 0.08
ff_prod_th = 350
ff_ratio_th = 0.75
[5]:
# Parameters
proposal = 2937
darkrun = 615
run = 614
module = 15
gain = 3.0
rois_th = 0.18
sat_level = 500
[6]:
params = boz.parameters(proposal=proposal, darkrun=darkrun, run=run, module=module, gain=gain, drop_intra_darks=drop_intra_darks)
[7]:
from extra_data.read_machinery import find_proposal
root = find_proposal(f'p{proposal:06d}')
path = root + f'/usr/processed_runs/r{params.run:04d}/'
print(path)
os.makedirs(path, exist_ok=True)
prefix = f'p{proposal}-r{run}-d{darkrun}-BOZ-Ia'
/gpfs/exfel/exp/SCS/202122/p002937/usr/processed_runs/r0614/
[8]:
print(params)
proposal:2937 darkrun:615 run:614 module:15 gain:3.0 ph/bin
drop intra darks:True
mask:None
rois threshold: None
rois: None
flat-field p: None prod:(5.0, inf) ratio:(-inf, 1.2)
plane guess fit: None
use hexagons: False
enforce mirror symmetry: True
ff alpha: None, max. iter.: None
Fnl: None
Load data persistently¶
[9]:
params.dask_load_persistently()
/gpfs/exfel/u/scratch/SCS/202301/p003423/envs/toolbox_p003423-gpu/lib/python3.9/site-packages/IPython/core/interactiveshell.py:3460: PerformanceWarning: Reshaping is producing a large chunk. To accept the large
chunk and silence this warning, set the option
>>> with dask.config.set(**{'array.slicing.split_large_chunks': False}):
... array.reshape(shape)
To avoid creating the large chunks, set the option
>>> with dask.config.set(**{'array.slicing.split_large_chunks': True}):
... array.reshape(shape)Explictly passing ``limit`` to ``reshape`` will also silence this warning
>>> array.reshape(shape, limit='128 MiB')
exec(code_obj, self.user_global_ns, self.user_ns)
/gpfs/exfel/u/scratch/SCS/202301/p003423/envs/toolbox_p003423-gpu/lib/python3.9/site-packages/IPython/core/interactiveshell.py:3460: PerformanceWarning: Reshaping is producing a large chunk. To accept the large
chunk and silence this warning, set the option
>>> with dask.config.set(**{'array.slicing.split_large_chunks': False}):
... array.reshape(shape)
To avoid creating the large chunks, set the option
>>> with dask.config.set(**{'array.slicing.split_large_chunks': True}):
... array.reshape(shape)Explictly passing ``limit`` to ``reshape`` will also silence this warning
>>> array.reshape(shape, limit='128 MiB')
exec(code_obj, self.user_global_ns, self.user_ns)
Slice the data by trainId if the number of pulses is large¶
[10]:
params.arr_dark
[10]:
|
[11]:
# keep only 1Gb of data
N = int(1*1024**3/(params.arr_dark.shape[1]*128*512*2))
SLICE = slice(0, N)
params.arr_dark = params.arr_dark[SLICE]
params.tid_dark = params.tid_dark[SLICE]
[12]:
params.arr
[12]:
|
[13]:
# keep only 4Gb of data
N = int(4*1024**3/(params.arr.shape[1]*128*512*2))
SLICE = slice(0, N)
params.arr = params.arr[SLICE]
params.tid = params.tid[SLICE]
[14]:
params.arr
[14]:
|
Use GPU¶
[15]:
dask.config.set(scheduler="single-threaded")
params.use_gpu()
Dark run inspection¶
The aim is to check dark level and extract bad pixel map.
[16]:
dark = boz.average_module(params.arr_dark).compute()
[17]:
pedestal = boz.ensure_on_host(np.mean(dark))
pedestal
[17]:
array(40.97503102)
[18]:
mean_th = (pedestal-12, pedestal+15)
f = boz.inspect_dark(boz.ensure_on_host(params.arr_dark),
mean_th=mean_th)
f.suptitle(f'p:{params.proposal} d:{params.darkrun}')
fname = path + prefix + '-inspect_dark.png'
f.savefig(fname, dpi=300)
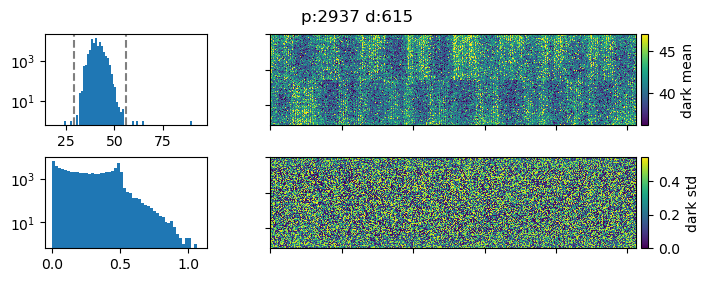
[19]:
params.mean_th = mean_th
params.set_mask(boz.bad_pixel_map(params))
# bad pixel: 12
[20]:
print(params)
proposal:2937 darkrun:615 run:614 module:15 gain:3.0 ph/bin
drop intra darks:True
mean threshold:(28.975031015633952, 55.97503101563395) std threshold:(None, None)
mask:(#12) [[15, 437], [36, 477], [43, 506], [59, 215], [61, 215], [63, 28], [71, 451], [76, 302], [80, 223], [80, 224], [108, 185], [125, 296]]
rois threshold: None
rois: None
flat-field p: None prod:(5.0, inf) ratio:(-inf, 1.2)
plane guess fit: None
use hexagons: False
enforce mirror symmetry: True
ff alpha: None, max. iter.: None
Fnl: None
Veto pattern check¶
Check potential veto pattern issue
[21]:
data = boz.average_module(params.arr, dark=dark).compute()
pp = boz.ensure_on_host(data.mean(axis=(1,2))) # pulseId resolved mean
dataM = boz.ensure_on_host(data.mean(axis=0)) # mean over pulseId
[22]:
plt.figure()
plt.plot(pp)
plt.xlabel('pulseId')
plt.ylabel('dark corrected module mean')
plt.title(f'p:{params.proposal} r:{params.run} d:{params.darkrun}')
plt.savefig(path+prefix+'-veto_inspect.png', dpi=300)
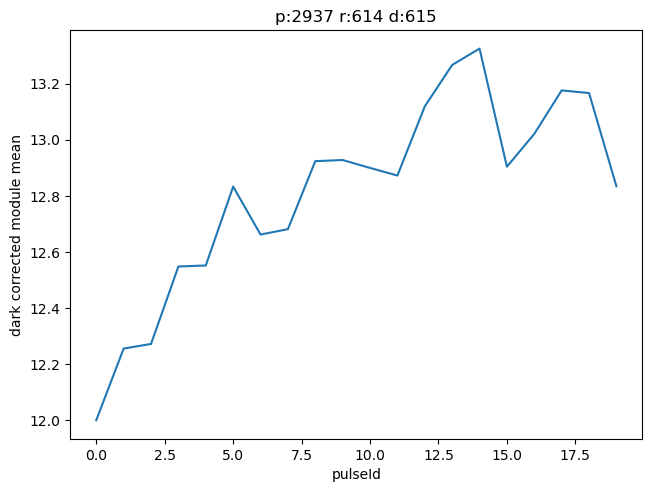
[23]:
# Thresholding out bad veto pulse
"""
threshold = 5
if False:
params.arr = params.arr[:, pp > threshold, :, :]
params.arr_dark = params.arr_dark[:, pp > threshold, :, :]
dark = boz.average_module(params.arr_dark).compute()
data = boz.average_module(params.arr, dark=dark).compute()
dataM = data.mean(axis=0) # mean over pulseId
"""
[23]:
'\nthreshold = 5\nif False:\n params.arr = params.arr[:, pp > threshold, :, :]\n params.arr_dark = params.arr_dark[:, pp > threshold, :, :]\n dark = boz.average_module(params.arr_dark).compute()\n data = boz.average_module(params.arr, dark=dark).compute()\n dataM = data.mean(axis=0) # mean over pulseId\n'
Histogram¶
[24]:
h, f = boz.inspect_histogram(boz.ensure_on_host(params.arr),
boz.ensure_on_host(params.arr_dark),
mask=boz.ensure_on_host(params.get_mask())
#, extra_lines=True
)
f.suptitle(f'p:{params.proposal} r:{params.run} d:{params.darkrun}')
f.savefig(path+prefix+'-histogram.png', dpi=300)
/home/lleguy/notebooks/ToolBox-git/src/toolbox_scs/routines/boz.py:598: PerformanceWarning: Reshaping is producing a large chunk. To accept the large
chunk and silence this warning, set the option
>>> with dask.config.set(**{'array.slicing.split_large_chunks': False}):
... array.reshape(shape)
To avoid creating the large chunks, set the option
>>> with dask.config.set(**{'array.slicing.split_large_chunks': True}):
... array.reshape(shape)Explictly passing ``limit`` to ``reshape`` will also silence this warning
>>> array.reshape(shape, limit='128 MiB')
h = ensure_on_host(histogram_module(arr, mask=mask))
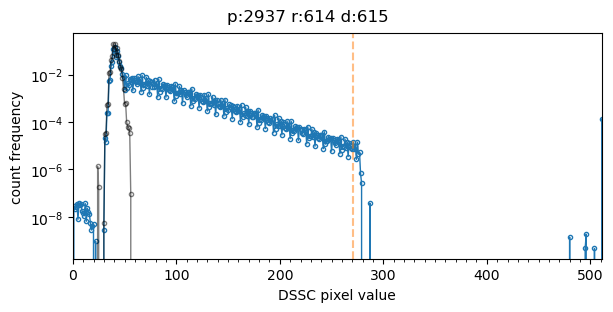
ROIs extraction¶
[25]:
params.rois_th = rois_th
params.rois = boz.find_rois_from_params(params)
[26]:
print(params)
proposal:2937 darkrun:615 run:614 module:15 gain:3.0 ph/bin
drop intra darks:True
mean threshold:(28.975031015633952, 55.97503101563395) std threshold:(None, None)
mask:(#12) [[15, 437], [36, 477], [43, 506], [59, 215], [61, 215], [63, 28], [71, 451], [76, 302], [80, 223], [80, 224], [108, 185], [125, 296]]
rois threshold: 0.18
rois: {'n': {'xl': 12, 'xh': 89, 'yl': 22, 'yh': 105}, '0': {'xl': 89, 'xh': 167, 'yl': 22, 'yh': 105}, 'p': {'xl': 167, 'xh': 244, 'yl': 22, 'yh': 105}, 'sat': {'xl': 12, 'xh': 244, 'yl': 22, 'yh': 105}}
flat-field p: None prod:(5.0, inf) ratio:(-inf, 1.2)
plane guess fit: None
use hexagons: False
enforce mirror symmetry: True
ff alpha: None, max. iter.: None
Fnl: None
[27]:
f = boz.inspect_rois(dataM, params.rois, params.rois_th)
f.savefig(path+prefix+f'-rois.png', dpi=300)
/home/lleguy/notebooks/ToolBox-git/src/toolbox_scs/routines/boz.py:767: MatplotlibDeprecationWarning: shading='flat' when X and Y have the same dimensions as C is deprecated since 3.3. Either specify the corners of the quadrilaterals with X and Y, or pass shading='auto', 'nearest' or 'gouraud', or set rcParams['pcolor.shading']. This will become an error two minor releases later.
main_ax.pcolormesh(Xs, Ys, np.flipud(data_mean[:, :256]),
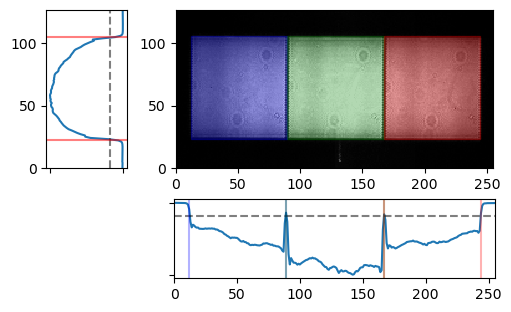
Flat field extraction¶
The first step is to compute a good average image, this mean remove saturated shots and ignoring bad pixels
[28]:
params.sat_level = sat_level
res = boz.average_module(params.arr, dark=dark,
ret='mean', mask=params.get_mask(), sat_roi=params.rois['sat'],
sat_level=params.sat_level)
avg = res.mean(axis=0).compute()
The second step is from that good average image to fit the plane field on n/0 and p/0 rois. We have to make sure that the rois have same width.
[29]:
# default values
params.flat_field_prod_th = (5.0, np.PINF)
params.flat_field_ratio_th = (np.NINF, 1.2)
params.use_hex = False
params.force_mirror = False
params.ff_alpha = 0.1
params.ff_max_iter = 25
print(params)
proposal:2937 darkrun:615 run:614 module:15 gain:3.0 ph/bin
drop intra darks:True
mean threshold:(28.975031015633952, 55.97503101563395) std threshold:(None, None)
mask:(#12) [[15, 437], [36, 477], [43, 506], [59, 215], [61, 215], [63, 28], [71, 451], [76, 302], [80, 223], [80, 224], [108, 185], [125, 296]]
rois threshold: 0.18
rois: {'n': {'xl': 12, 'xh': 89, 'yl': 22, 'yh': 105}, '0': {'xl': 89, 'xh': 167, 'yl': 22, 'yh': 105}, 'p': {'xl': 167, 'xh': 244, 'yl': 22, 'yh': 105}, 'sat': {'xl': 12, 'xh': 244, 'yl': 22, 'yh': 105}}
flat-field p: None prod:(5.0, inf) ratio:(-inf, 1.2)
plane guess fit: None
use hexagons: False
enforce mirror symmetry: False
ff alpha: 0.1, max. iter.: 25
Fnl: None
Refining flat field¶
[30]:
res, cb = boz.ff_refine_fit(params)
0: 0:00:00.000001 (18.4836060294627, 95.86388810686377, 792.2864268034733), [-0.2, -0.1, 1, -0.54, 0.2, -0.1, 1, -0.54]
1: 0:00:14.281071 (1.704098033186518, 74.25427490721434, 727.2058667734647), [-0.2058043 -0.0792378 1.00268546 -0.53672204 0.20203086 -0.09360214
0.97615395 -0.58459197]
2: 0:00:18.655748 (11.890682695560002, 61.75454580368649, 510.5293137768249), [-0.22922627 -0.00316114 0.97621938 -0.58932566 0.21332385 -0.07818025
0.96348728 -0.60881437]
3: 0:00:23.169202 (1.9664154222668786, 54.483411566268536, 527.1363768622834), [-0.26789167 0.08104171 0.991437 -0.5666215 0.23390899 -0.02913895
0.97200164 -0.59716311]
4: 0:00:30.855880 (2.788852315822956, 51.780828173217316, 492.7086108897666), [-0.28686793 0.08023693 0.99309416 -0.5720163 0.24892017 0.0086582
0.9721102 -0.59781099]
5: 0:00:35.233928 (2.8306980133010997, 46.731008152166524, 441.83379940195533), [-0.33479122 0.073223 1.00089164 -0.57662417 0.2996213 0.01636881
0.96678814 -0.59674371]
6: 0:00:39.613031 (1.1027609383335018, 41.17392391325677, 401.8143906875661), [-0.42657317 0.07059582 1.41240491 -0.79234128 0.29854325 0.01741615
0.8727304 -0.5401786 ]
7: 0:00:47.271427 (0.3834769179951083, 40.067238110104064, 397.22108883908464), [-0.34358714 0.0842115 1.49996193 -0.83727507 0.39646279 0.02845304
0.8808928 -0.54309903]
8: 0:00:55.050496 (0.4623140913753959, 31.66381594753332, 312.47733265295466), [-0.56869177 0.03071662 1.43181094 -0.80317794 0.37921352 0.00833382
0.93026185 -0.5700529 ]
9: 0:01:02.729413 (0.5262076728723595, 31.533228320912848, 310.59641415327724), [-0.56596605 0.03091362 1.42287508 -0.79821606 0.38315469 0.01139978
0.89660161 -0.55178684]
10: 0:01:10.393711 (0.40577343836681873, 30.558773621350866, 301.9357752682073), [-0.60374124 0.01543549 1.40900101 -0.79059803 0.38721309 0.00676208
0.92332579 -0.56538049]
11: 0:01:18.169954 (0.29536178209128877, 30.14022919095299, 298.74403587070833), [-0.59873252 0.01126187 1.47905137 -0.82735865 0.41319924 0.00458773
0.91818125 -0.56084676]
12: 0:01:22.553112 (0.23277723937779787, 29.98455620216637, 297.7505668672635), [-0.62677837 -0.00429811 1.46602808 -0.81904516 0.41077136 0.00368263
0.95237687 -0.57894441]
13: 0:01:26.940119 (0.1677490401115567, 29.89336741828346, 297.4239328218306), [-6.20967484e-01 4.92248974e-04 1.48469078e+00 -8.27985442e-01
4.08252795e-01 2.84273532e-03 9.38604555e-01 -5.71401832e-01]
14: 0:01:31.327848 (0.12004223960690431, 29.88067993575002, 297.726419201038), [-6.20858884e-01 1.11764583e-03 1.49346862e+00 -8.31514626e-01
4.06801459e-01 3.23657214e-03 9.32085950e-01 -5.67486100e-01]
15: 0:01:35.715057 (0.08534285020804122, 29.876609006905095, 297.99800441717855), [-6.22344600e-01 7.21760918e-04 1.49956749e+00 -8.33734042e-01
4.04782012e-01 3.07071239e-03 9.29454644e-01 -5.65835079e-01]
16: 0:01:40.104267 (0.08380257947297409, 29.87653945624043, 298.0111713471475), [-6.22203211e-01 7.85738897e-04 1.50051239e+00 -8.34194153e-01
4.05073545e-01 3.25776827e-03 9.29479050e-01 -5.65778142e-01]
17: 0:01:44.495705 (0.08425002000016209, 29.876523092452047, 298.006980744519), [-6.22301296e-01 7.26689476e-04 1.50018252e+00 -8.34031747e-01
4.05003387e-01 3.19353498e-03 9.29539604e-01 -5.65831164e-01]
18: 0:01:48.998946 (0.08427220167345736, 29.87652308110631, 298.00678099600196), [-6.22303819e-01 7.24886121e-04 1.50017694e+00 -8.34029572e-01
4.05002711e-01 3.19264427e-03 9.29552380e-01 -5.65838672e-01]
19: 0:01:53.396445 (0.08427383467812025, 29.87652308099577, 298.0067662978546), [-6.22303994e-01 7.24728531e-04 1.50017648e+00 -8.34029378e-01
4.05002952e-01 3.19271474e-03 9.29552217e-01 -5.65838631e-01]
20: 0:01:57.800381 (0.08427416822406841, 29.876523080994907, 298.00676329593244), [-6.22304067e-01 7.24733676e-04 1.50017671e+00 -8.34029520e-01
4.05003057e-01 3.19272047e-03 9.29552429e-01 -5.65838772e-01]
21: 0:02:22.053462 (0.08427417717855114, 29.87652308099486, 298.00676321534166), [-6.22304070e-01 7.24733782e-04 1.50017672e+00 -8.34029526e-01
4.05003061e-01 3.19272061e-03 9.29552438e-01 -5.65838778e-01]
Warning: Desired error not necessarily achieved due to precision loss.
Current function value: 29.876523
Iterations: 21
Function evaluations: 651
Gradient evaluations: 71
[31]:
params.set_flat_field(res.x)
[32]:
ff = boz.compute_flat_field_correction(params.rois, params)
f = boz.inspect_plane_fitting(boz.ensure_on_host(avg)/ff, params.rois)
f.savefig(path+prefix+'-inspect-withflatfield-refined.png', dpi=300)
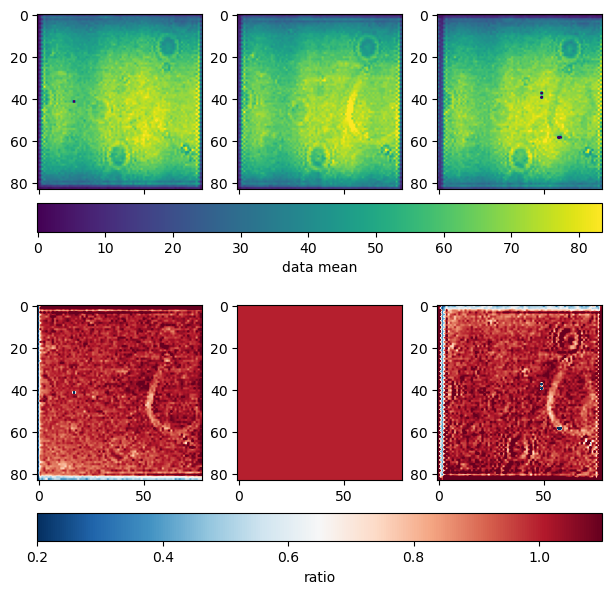
Non-linearities correction extraction¶
To speed up online analysis, we save the corrections with a dummy non-linearity correction. The saved file can be used for online analysis as soon as it appears.
[33]:
params.set_Fnl(np.arange(2**9))
params.save(path=path)
/gpfs/exfel/exp/SCS/202122/p002937/usr/processed_runs/r0614/parameters_p2937_d615_r614.json
[34]:
N = 80
domain = boz.nl_domain(N, 40, 511)
params.nl_alpha = 0.5
params.nl_max_iter = 25
minimizing¶
[35]:
res, fit_res = boz.nl_fit(params, domain)
0: 0:00:00.000001 (3086.0699271427047, 1543.0349635713524, 0.0), [0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0 0
0 0 0 0 0 0]
1: 0:00:58.777819 (1486.0545571870425, 746.0852336389034, 6.115910090764297), [-1.57636853e-01 -4.99656139e-01 -4.12595060e-02 4.91009113e-01
4.91730605e-01 3.52349007e-01 9.33380898e-02 -4.54187036e-02
-1.40888962e-01 -1.91877987e-01 -1.62450269e-01 -1.31042085e-01
-1.10913045e-01 -8.06552446e-02 -4.61741230e-02 -3.09095183e-02
-1.50260207e-02 8.84763575e-04 1.29982137e-02 1.83006270e-02
2.49125057e-02 2.18491230e-02 2.26397447e-02 2.27667555e-02
2.00809841e-02 1.76044770e-02 1.32664669e-02 9.73182337e-03
8.40726981e-03 4.19531553e-03 3.66141318e-03 2.65210996e-03
1.38896229e-03 9.11011464e-04 4.56409049e-04 2.92753558e-04
1.26513645e-04 7.69714922e-05 3.40700753e-05 1.52505553e-05
1.91493415e-06 0.00000000e+00 0.00000000e+00 -1.01063234e-05
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
2: 0:01:57.609617 (1240.9171005959702, 624.2149507031482, 7.512800810326353), [-2.68572233e-01 -3.59878600e-01 6.13434905e-02 5.44656994e-01
5.59683714e-01 4.31540425e-01 1.32802698e-01 -3.87940851e-02
-1.64732311e-01 -2.43392693e-01 -2.18942805e-01 -1.86267432e-01
-1.66367657e-01 -1.28463788e-01 -7.84566497e-02 -5.69307934e-02
-3.33554472e-02 -5.89918240e-03 1.42538832e-02 2.53876360e-02
3.73202443e-02 3.40889060e-02 3.62029912e-02 3.71500569e-02
3.33268366e-02 2.96272520e-02 2.25824185e-02 1.67072406e-02
1.45343795e-02 7.28336187e-03 6.38451902e-03 4.63892280e-03
2.43546781e-03 1.60011808e-03 8.02811463e-04 5.15876609e-04
2.22283562e-04 1.36017344e-04 6.02644228e-05 2.69936443e-05
3.38215057e-06 0.00000000e+00 0.00000000e+00 -2.50418059e-05
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
3: 0:02:27.605019 (1188.6933603905436, 599.7408627633871, 10.788365136230587), [-3.66103193e-01 -2.41082143e-01 8.88901871e-03 4.20239863e-01
6.50507362e-01 6.67516377e-01 3.32529601e-01 1.03993048e-01
-7.93325789e-02 -2.38019112e-01 -2.75938308e-01 -2.76103162e-01
-2.82475433e-01 -2.48632339e-01 -1.72043232e-01 -1.43336354e-01
-1.07869874e-01 -4.80045075e-02 -8.99119466e-03 2.33953614e-02
5.01926834e-02 5.29023771e-02 6.05116348e-02 6.56145813e-02
6.14280326e-02 5.64519958e-02 4.41547984e-02 3.32822761e-02
2.93886864e-02 1.48774246e-02 1.31438466e-02 9.61091881e-03
5.07136364e-03 3.34349883e-03 1.68252312e-03 1.08510075e-03
4.66209884e-04 2.87771271e-04 1.27765101e-04 5.73075757e-05
7.13309594e-06 0.00000000e+00 0.00000000e+00 -6.35821915e-05
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
4: 0:02:57.603065 (1122.0802050307889, 567.0397227755255, 11.999240520262267), [-4.30698411e-01 -2.64088684e-01 6.79413360e-02 4.50754533e-01
6.53790771e-01 6.68920117e-01 3.57948163e-01 1.59563570e-01
5.99242732e-03 -1.52485361e-01 -2.33082161e-01 -2.72519107e-01
-3.14069488e-01 -3.06914620e-01 -2.31961482e-01 -2.09647014e-01
-1.75921993e-01 -9.47186780e-02 -4.47931942e-02 7.17095673e-03
4.58886573e-02 5.79868364e-02 7.15882992e-02 8.17682700e-02
7.95224136e-02 7.52011920e-02 6.01092048e-02 4.60088009e-02
4.11231001e-02 2.09905592e-02 1.86603458e-02 1.37139623e-02
7.26591147e-03 4.80348268e-03 2.42292542e-03 1.56709615e-03
6.73614707e-04 4.17571507e-04 1.85679029e-04 8.33680182e-05
1.03205279e-05 0.00000000e+00 0.00000000e+00 -1.02947069e-04
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
5: 0:03:27.660158 (1089.3173545649247, 552.5483584425975, 15.77936232027031), [-4.53231662e-01 -1.17850414e-01 -2.44914618e-02 5.36071713e-01
8.63433458e-01 7.24940773e-01 2.84426055e-01 1.10783010e-01
6.36224003e-02 2.50042930e-02 -5.63200346e-02 -1.53353607e-01
-2.68707692e-01 -3.54916858e-01 -3.33950806e-01 -3.58109121e-01
-3.60986870e-01 -2.43352541e-01 -1.78089145e-01 -6.98630401e-02
1.00751077e-03 5.02113131e-02 8.50937013e-02 1.15123333e-01
1.24942037e-01 1.27589573e-01 1.07820862e-01 8.57326135e-02
7.89464888e-02 4.11164424e-02 3.70965436e-02 2.75925060e-02
1.47628506e-02 9.82099953e-03 4.98035333e-03 3.24255144e-03
1.39877304e-03 8.73461294e-04 3.89512545e-04 1.75193469e-04
2.13487740e-05 0.00000000e+00 0.00000000e+00 -2.81134327e-04
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
6: 0:03:57.859446 (1063.4484495422125, 541.3134813437672, 19.17851314532177), [-4.22747617e-01 -1.33796521e-01 1.39159522e-01 4.78754134e-01
9.06581143e-01 8.73126937e-01 3.64583287e-01 1.00330310e-01
4.10943791e-03 1.18520737e-02 2.28042724e-02 -2.54886843e-02
-1.19173107e-01 -2.52747545e-01 -3.17906931e-01 -4.11410296e-01
-4.90874442e-01 -3.87614912e-01 -3.39517046e-01 -1.87181698e-01
-9.47413435e-02 3.57511361e-03 6.40948846e-02 1.21878076e-01
1.55616919e-01 1.75351363e-01 1.58233847e-01 1.31270123e-01
1.24881807e-01 6.65210505e-02 6.09021397e-02 4.58669045e-02
2.47950820e-02 1.65965991e-02 8.45996263e-03 5.54396041e-03
2.39950028e-03 1.50953597e-03 6.74237808e-04 3.03593361e-04
3.63396857e-05 0.00000000e+00 0.00000000e+00 -6.20640043e-04
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
7: 0:04:27.900157 (1030.0318523499377, 526.5092541875989, 22.98665602526022), [-2.08136666e-01 -6.37634014e-02 2.15604662e-01 6.08830771e-01
9.33237150e-01 8.80906758e-01 4.71595009e-01 2.23984119e-01
5.72674353e-02 -3.23997482e-02 -2.11437174e-02 -2.17730850e-02
-4.92873752e-02 -1.60892819e-01 -2.74067729e-01 -4.17975420e-01
-5.70015441e-01 -5.03147458e-01 -4.85727527e-01 -3.04495795e-01
-2.00485292e-01 -5.46222323e-02 3.08481454e-02 1.18823306e-01
1.82361771e-01 2.25275464e-01 2.15036656e-01 1.84640133e-01
1.80315938e-01 9.79144032e-02 9.05513139e-02 6.88610553e-02
3.75310753e-02 2.52357195e-02 1.29109109e-02 8.50192328e-03
3.69289737e-03 2.33335980e-03 1.04205834e-03 4.69314699e-04
5.52322272e-05 0.00000000e+00 0.00000000e+00 -1.16780373e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
8: 0:04:57.925529 (977.4904014781654, 502.358517727857, 27.226633977548637), [-1.82242260e-01 -3.32384226e-02 3.30859429e-01 5.58146899e-01
1.16277261e+00 8.73385361e-01 4.22962085e-01 3.28710375e-01
2.49557516e-01 6.09801552e-02 -7.03252970e-02 -9.82146283e-02
-3.12495956e-02 -2.36712722e-02 -1.30147411e-01 -2.83129610e-01
-4.96248266e-01 -5.29547670e-01 -5.83778232e-01 -4.25411818e-01
-3.50146294e-01 -1.68254077e-01 -6.65623617e-02 5.15685011e-02
1.61170766e-01 2.46189289e-01 2.62317124e-01 2.39593698e-01
2.45010241e-01 1.38264257e-01 1.29366414e-01 1.00007211e-01
5.52700462e-02 3.74348035e-02 1.92575687e-02 1.27796056e-02
5.61436203e-03 3.55157636e-03 1.58216133e-03 7.11430135e-04
8.05375982e-05 0.00000000e+00 0.00000000e+00 -2.40478943e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
9: 0:05:27.980489 (888.7621864423633, 458.8727035210133, 28.983220599663355), [-1.99547815e-02 1.76559243e-02 4.51396953e-01 6.32561460e-01
1.14454695e+00 1.03243310e+00 4.97479845e-01 2.77336275e-01
2.59482720e-01 2.61309675e-01 8.64630076e-02 -1.02405282e-01
-1.02579621e-01 -5.42087480e-02 -9.79864156e-02 -1.88978158e-01
-3.54486930e-01 -4.36442804e-01 -5.12640848e-01 -4.09401063e-01
-3.86260018e-01 -2.34988580e-01 -1.44642763e-01 -3.02618780e-02
1.05284957e-01 2.24027480e-01 2.72826189e-01 2.66648126e-01
2.87235730e-01 1.72208067e-01 1.60391320e-01 1.26318531e-01
7.09723904e-02 4.84251860e-02 2.50249040e-02 1.67456773e-02
7.55921543e-03 4.71215748e-03 2.07434799e-03 9.28190008e-04
9.78009558e-05 0.00000000e+00 0.00000000e+00 -4.72443512e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
10: 0:05:58.030965 (757.9851791689786, 394.6062792015764, 31.227379234174247), [-3.50308804e-01 1.26897028e-01 2.42826447e-01 8.83511882e-01
9.51962300e-01 1.01250413e+00 8.24969491e-01 4.03914077e-01
1.96983351e-01 3.51026963e-01 3.20118926e-01 6.19688067e-02
1.68637204e-02 5.06061237e-02 1.29467694e-04 -5.93062614e-02
-1.87242460e-01 -3.34080827e-01 -4.28403122e-01 -3.84171388e-01
-4.22185066e-01 -3.21422473e-01 -2.41298541e-01 -1.41836307e-01
1.74528956e-02 1.70953919e-01 2.60433381e-01 2.77877967e-01
3.18310054e-01 2.07819481e-01 1.88779208e-01 1.51535384e-01
8.66188719e-02 5.95017490e-02 3.08532158e-02 2.08084208e-02
9.81492511e-03 5.91582057e-03 2.55698874e-03 1.13648936e-03
1.07390344e-04 0.00000000e+00 0.00000000e+00 -8.44204665e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
11: 0:06:28.079323 (611.025588374486, 318.7935051642118, 26.561421953937607), [-2.11920251e-01 -8.22691516e-02 3.37851577e-01 2.80009473e-01
1.37813473e+00 1.20348629e-01 5.79705338e-01 6.92884309e-01
4.83428467e-03 -4.23393437e-01 4.21314810e-02 2.88451303e-01
2.45908544e-01 -1.23694250e-01 -3.66681346e-01 -5.60545393e-01
-5.92164691e-01 -4.19763757e-01 -2.34963179e-01 -1.71214657e-02
1.14663562e-01 8.83223923e-02 1.94021922e-01 1.91814586e-01
1.72463656e-01 1.48760729e-01 1.15535040e-01 8.68372927e-02
8.38938581e-02 8.68385064e-02 4.43812134e-02 3.51593657e-02
2.06677091e-02 1.38707197e-02 6.86306376e-03 4.59845998e-03
3.88370023e-03 1.22854638e-03 3.39460519e-04 1.23546506e-04
-2.84520108e-05 0.00000000e+00 0.00000000e+00 -9.29615251e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
12: 0:07:27.445749 (526.3965271898231, 281.9141169232144, 37.43170665660572), [-4.28574427e-01 -3.01939921e-01 -1.05248375e-01 1.50263755e-01
2.72515112e-01 5.10997855e-01 -5.39809563e-01 -7.11888277e-02
1.48121593e-01 -6.34020527e-01 -8.79080825e-01 -3.91122961e-01
-9.64832942e-02 -2.11023347e-01 -4.26040360e-01 -7.58660949e-01
-1.05448123e+00 -8.43599610e-01 -7.78742630e-01 -4.13695361e-01
-1.32449407e-01 1.10140792e-01 3.01685886e-01 4.15648773e-01
3.89837437e-01 2.99172820e-01 1.57192493e-01 5.78031538e-02
-1.04300838e-02 -1.25925721e-02 -4.76279601e-02 -4.80662902e-02
-3.16711981e-02 -2.33770177e-02 -1.27943476e-02 -9.18687415e-03
-2.79776084e-03 -2.89135718e-03 -1.31499475e-03 -5.86178352e-04
-5.96830027e-05 0.00000000e+00 0.00000000e+00 1.41500551e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
13: 0:07:57.509786 (451.5572067764707, 246.68270049959162, 41.80819422271255), [-2.71639785e-01 -2.74471859e-01 4.63203077e-02 2.41993437e-02
6.31128460e-01 -1.81741861e-01 3.96920047e-01 -3.72745532e-01
-5.87532755e-01 -1.86668367e-01 -3.59265687e-01 -6.61061477e-01
-7.99887781e-01 -5.71673879e-01 -4.58427729e-01 -4.81624390e-01
-8.01880564e-01 -7.70770710e-01 -9.51238900e-01 -7.77301035e-01
-7.22922329e-01 -3.47924947e-01 -3.52501047e-01 -1.77723573e-01
-9.40529337e-04 1.60161647e-01 2.37431906e-01 2.51620159e-01
2.70602856e-01 8.51544868e-02 1.47896830e-01 1.14716738e-01
6.18684230e-02 4.25683609e-02 2.26373673e-02 1.50800132e-02
3.23809814e-03 4.37899547e-03 2.40124671e-03 1.14088281e-03
2.21144245e-04 0.00000000e+00 0.00000000e+00 1.17607666e-02
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
14: 0:08:56.712505 (442.1896165874871, 230.28499118125276, 18.380365775018426), [-5.94735802e-02 1.19482034e-03 3.71908465e-01 4.49464956e-01
8.10193555e-01 5.72875618e-01 3.68055311e-01 1.96176050e-01
1.87500458e-01 -5.80690442e-03 2.10595046e-02 -8.63783551e-02
-1.59367655e-01 -2.17250813e-01 -3.20416856e-01 -2.52726431e-01
-3.24347917e-01 -3.75528416e-01 -4.62313062e-01 -4.16560432e-01
-4.54656874e-01 -3.57303480e-01 -2.82545076e-01 -1.91997331e-01
-4.72829853e-02 9.60931373e-02 1.90163508e-01 2.21073489e-01
2.64588909e-01 1.44756342e-01 1.64303853e-01 1.34013559e-01
7.71545473e-02 5.36060848e-02 2.81205490e-02 1.90774885e-02
7.26613089e-03 5.52515436e-03 2.53453782e-03 1.14546128e-03
1.37574139e-04 0.00000000e+00 0.00000000e+00 -9.57968283e-05
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
15: 0:09:55.741792 (442.55772594069373, 229.90635865183347, 17.254991362973197), [-5.75971085e-02 9.71157523e-03 3.81207565e-01 4.64101877e-01
8.26154540e-01 5.82995314e-01 4.07081987e-01 2.29964435e-01
1.24225457e-01 1.33602082e-01 3.77475648e-02 -1.54953446e-01
-1.64069673e-01 -1.14271520e-01 -1.85883393e-01 -2.15872633e-01
-3.44938514e-01 -4.08348113e-01 -4.60246062e-01 -3.69982339e-01
-3.70745692e-01 -2.90722528e-01 -1.89080410e-01 -1.04940791e-01
1.83993865e-02 1.33324822e-01 1.97964369e-01 2.09153044e-01
2.38087643e-01 1.51697659e-01 1.40531330e-01 1.12725700e-01
6.44092906e-02 4.42532017e-02 2.29508661e-02 1.54706539e-02
7.21807464e-03 4.39393538e-03 1.90458150e-03 8.45804386e-04
8.22702684e-05 0.00000000e+00 0.00000000e+00 -4.93948082e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
16: 0:10:54.781969 (442.70188344589843, 229.87218627370297, 17.04248910150751), [-6.58721316e-02 8.17370651e-03 3.76028800e-01 4.63618398e-01
8.23455702e-01 5.84342987e-01 4.01330380e-01 2.34012482e-01
1.34993820e-01 1.18353970e-01 3.78834289e-02 -1.21835242e-01
-1.63787701e-01 -1.52227027e-01 -1.43428284e-01 -2.13685594e-01
-3.25284463e-01 -3.99082442e-01 -4.67288808e-01 -3.80399202e-01
-3.74678750e-01 -2.64097514e-01 -1.69107489e-01 -7.86351584e-02
4.34455806e-02 1.52631907e-01 2.08535386e-01 2.13153949e-01
2.37820846e-01 1.64659392e-01 1.37517396e-01 1.09512203e-01
6.22921378e-02 4.26021546e-02 2.19999127e-02 1.47865635e-02
7.65258553e-03 4.17455938e-03 1.77943087e-03 7.88052116e-04
7.05183673e-05 0.00000000e+00 0.00000000e+00 -8.55302894e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
17: 0:11:24.842809 (442.88782239485795, 229.83843273571858, 16.789043076579198), [-7.86656716e-02 7.69939304e-03 3.68956799e-01 4.65456855e-01
8.18497482e-01 5.90733322e-01 3.90724392e-01 2.36730322e-01
1.49925745e-01 1.14108100e-01 2.09059679e-02 -1.26906022e-01
-1.34312501e-01 -1.28452185e-01 -1.50203782e-01 -2.41151336e-01
-3.47024572e-01 -3.96304240e-01 -4.46599829e-01 -3.61558422e-01
-3.62540297e-01 -2.57719723e-01 -1.73720927e-01 -8.63186951e-02
3.57839902e-02 1.46237866e-01 2.04242962e-01 2.10553335e-01
2.36196545e-01 1.67062694e-01 1.37393576e-01 1.09691333e-01
6.25063679e-02 4.28262970e-02 2.21493178e-02 1.48998909e-02
7.74890377e-03 4.21270327e-03 1.80322214e-03 7.97686999e-04
7.21975587e-05 0.00000000e+00 0.00000000e+00 -9.27368110e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
18: 0:12:23.875630 (442.921245449471, 229.83468615095325, 16.74812685243551), [-8.01093743e-02 7.88368564e-03 3.68280488e-01 4.66091447e-01
8.17476063e-01 5.93595190e-01 3.84183590e-01 2.45210065e-01
1.48329715e-01 1.08673099e-01 2.82574697e-02 -1.26471534e-01
-1.39477123e-01 -1.22427950e-01 -1.64734388e-01 -2.27476149e-01
-3.44098333e-01 -4.03350319e-01 -4.54237652e-01 -3.62545906e-01
-3.57084869e-01 -2.63645320e-01 -1.67453686e-01 -8.29497092e-02
3.51790903e-02 1.42441301e-01 1.99383828e-01 2.06022216e-01
2.31798967e-01 1.59198572e-01 1.35382971e-01 1.08255496e-01
6.17746232e-02 4.23477747e-02 2.19071409e-02 1.47471519e-02
7.44987178e-03 4.17294738e-03 1.78716348e-03 7.89975290e-04
7.26470781e-05 0.00000000e+00 0.00000000e+00 -7.65163072e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
19: 0:13:22.988183 (442.92547055591086, 229.83458975919572, 16.743708962480607), [-8.01228164e-02 7.85617819e-03 3.68280852e-01 4.66017877e-01
8.17691400e-01 5.93033826e-01 3.85497394e-01 2.43708751e-01
1.48697482e-01 1.09754977e-01 2.65641478e-02 -1.25959083e-01
-1.38534190e-01 -1.22283099e-01 -1.66710459e-01 -2.26794493e-01
-3.43025451e-01 -4.02824467e-01 -4.54319174e-01 -3.62904427e-01
-3.57331794e-01 -2.65442484e-01 -1.66513699e-01 -8.16264783e-02
3.64795303e-02 1.43366603e-01 1.99734919e-01 2.05948742e-01
2.31404194e-01 1.57457509e-01 1.34962077e-01 1.07869107e-01
6.15381387e-02 4.21761514e-02 2.18115537e-02 1.46802362e-02
7.37514163e-03 4.15288590e-03 1.77627007e-03 7.85665090e-04
7.13405621e-05 0.00000000e+00 0.00000000e+00 -7.31245956e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
20: 0:14:22.050028 (442.9256335662375, 229.83457951935975, 16.743525472482048), [-8.01074332e-02 7.73854829e-03 3.68183213e-01 4.65866447e-01
8.17474815e-01 5.92955396e-01 3.85150365e-01 2.43723868e-01
1.48388668e-01 1.09535979e-01 2.66000932e-02 -1.26006504e-01
-1.39075455e-01 -1.22626772e-01 -1.66250098e-01 -2.26855098e-01
-3.43313715e-01 -4.02956643e-01 -4.54312412e-01 -3.62816843e-01
-3.57306282e-01 -2.66253880e-01 -1.66520252e-01 -8.15478359e-02
3.67315417e-02 1.43768706e-01 2.00132768e-01 2.06263644e-01
2.31643640e-01 1.56622147e-01 1.35008019e-01 1.07871647e-01
6.15255381e-02 4.21597609e-02 2.18007622e-02 1.46704484e-02
7.33798602e-03 4.14800245e-03 1.77232589e-03 7.84703805e-04
7.13316352e-05 0.00000000e+00 0.00000000e+00 -7.13532471e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
21: 0:14:52.122790 (442.92622105130977, 229.83457222022807, 16.74292338914637), [-8.00202484e-02 7.61243743e-03 3.68111842e-01 4.65694212e-01
8.17258847e-01 5.92758149e-01 3.85125119e-01 2.43181907e-01
1.48225947e-01 1.09511781e-01 2.63560783e-02 -1.26355145e-01
-1.39409454e-01 -1.23019658e-01 -1.66317959e-01 -2.26686944e-01
-3.43154203e-01 -4.03253790e-01 -4.55097785e-01 -3.63446977e-01
-3.57555039e-01 -2.65666124e-01 -1.66318047e-01 -8.13935932e-02
3.66430001e-02 1.43475602e-01 1.99806907e-01 2.06007023e-01
2.31447224e-01 1.56990209e-01 1.34975769e-01 1.07873254e-01
6.15379346e-02 4.21722733e-02 2.18088889e-02 1.46793810e-02
7.35382266e-03 4.15295645e-03 1.77714763e-03 7.86973740e-04
7.04564700e-05 0.00000000e+00 0.00000000e+00 -7.21542959e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
22: 0:15:51.534856 (442.9263038626892, 229.83457168941098, 16.74283951613272), [-8.00308336e-02 7.60088489e-03 3.68097512e-01 4.65680601e-01
8.17242908e-01 5.92756923e-01 3.85064735e-01 2.43257213e-01
1.48154881e-01 1.09507896e-01 2.63701478e-02 -1.26337729e-01
-1.39460505e-01 -1.22922608e-01 -1.66470214e-01 -2.26628535e-01
-3.43161881e-01 -4.03211949e-01 -4.54989741e-01 -3.63408301e-01
-3.57640473e-01 -2.65628434e-01 -1.66399240e-01 -8.13832979e-02
3.67530721e-02 1.43630984e-01 1.99923973e-01 2.06066283e-01
2.31460898e-01 1.57167643e-01 1.34944854e-01 1.07837225e-01
6.15125369e-02 4.21553888e-02 2.17984922e-02 1.46712694e-02
7.36099092e-03 4.14989065e-03 1.77567571e-03 7.84431509e-04
7.10523579e-05 0.00000000e+00 0.00000000e+00 -7.25290720e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
23: 0:16:21.768297 (442.92614847467667, 229.8345713195211, 16.742994164365566), [-8.00517912e-02 7.62697997e-03 3.68110588e-01 4.65718230e-01
8.17291669e-01 5.92786562e-01 3.85115782e-01 2.43298565e-01
1.48248757e-01 1.09495750e-01 2.64191861e-02 -1.26254945e-01
-1.39387061e-01 -1.22863287e-01 -1.66412960e-01 -2.26625810e-01
-3.43219594e-01 -4.03183677e-01 -4.54858379e-01 -3.63230273e-01
-3.57502792e-01 -2.65803848e-01 -1.66398386e-01 -8.14414291e-02
3.67335295e-02 1.43659615e-01 1.99989279e-01 2.06132305e-01
2.31536124e-01 1.57103940e-01 1.34974038e-01 1.07869171e-01
6.15256688e-02 4.21630823e-02 2.18026435e-02 1.46747185e-02
7.36239245e-03 4.15295744e-03 1.77000985e-03 7.91071812e-04
7.76745966e-05 0.00000000e+00 0.00000000e+00 -7.23708698e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
24: 0:17:20.876679 (442.92613151978617, 229.83457126777625, 16.74301101576635), [-8.00504605e-02 7.63158019e-03 3.68115028e-01 4.65724350e-01
8.17296382e-01 5.92798854e-01 3.85097399e-01 2.43348603e-01
1.48216549e-01 1.09516180e-01 2.64243301e-02 -1.26262656e-01
-1.39369575e-01 -1.22862792e-01 -1.66406179e-01 -2.26673272e-01
-3.43199728e-01 -4.03156687e-01 -4.54841974e-01 -3.63261010e-01
-3.57541461e-01 -2.65797407e-01 -1.66415868e-01 -8.14295427e-02
3.67354874e-02 1.43650636e-01 1.99970413e-01 2.06115597e-01
2.31513982e-01 1.57051727e-01 1.34967377e-01 1.07854745e-01
6.15203833e-02 4.21601706e-02 2.18012491e-02 1.46733476e-02
7.35782828e-03 4.15011235e-03 1.77358434e-03 7.86479355e-04
7.21457653e-05 0.00000000e+00 0.00000000e+00 -7.22671285e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
25: 0:17:50.966772 (442.92620525360365, 229.83457125323253, 16.742937252861374), [-8.00453334e-02 7.62612083e-03 3.68112793e-01 4.65716488e-01
8.17287237e-01 5.92789810e-01 3.85099098e-01 2.43318538e-01
1.48217035e-01 1.09508744e-01 2.64204350e-02 -1.26275695e-01
-1.39399786e-01 -1.22860948e-01 -1.66420706e-01 -2.26657858e-01
-3.43191302e-01 -4.03181635e-01 -4.54859594e-01 -3.63298806e-01
-3.57576383e-01 -2.65715514e-01 -1.66431840e-01 -8.14228426e-02
3.67432700e-02 1.43658157e-01 1.99958967e-01 2.06103849e-01
2.31494991e-01 1.57034560e-01 1.34961857e-01 1.07848008e-01
6.15195621e-02 4.21554264e-02 2.18073533e-02 1.46723419e-02
7.35637424e-03 4.15214780e-03 1.78038360e-03 7.85856408e-04
7.76728798e-05 0.00000000e+00 0.00000000e+00 -7.22107260e-03
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
Warning: Maximum number of iterations has been exceeded.
Current function value: 229.834571
Iterations: 25
Function evaluations: 2916
Gradient evaluations: 36
[36]:
params.set_Fnl(boz.nl_lut(domain, res.x))
[37]:
print(params)
proposal:2937 darkrun:615 run:614 module:15 gain:3.0 ph/bin
drop intra darks:True
mean threshold:(28.975031015633952, 55.97503101563395) std threshold:(None, None)
mask:(#12) [[15, 437], [36, 477], [43, 506], [59, 215], [61, 215], [63, 28], [71, 451], [76, 302], [80, 223], [80, 224], [108, 185], [125, 296]]
rois threshold: 0.18
rois: {'n': {'xl': 12, 'xh': 89, 'yl': 22, 'yh': 105}, '0': {'xl': 89, 'xh': 167, 'yl': 22, 'yh': 105}, 'p': {'xl': 167, 'xh': 244, 'yl': 22, 'yh': 105}, 'sat': {'xl': 12, 'xh': 244, 'yl': 22, 'yh': 105}}
flat-field p: [-0.6223040701072852, 0.000724733782145572, 1.500176720279694, -0.8340295260244752, 0.4050030612762583, 0.003192720612202234, 0.9295524383664061, -0.5658387778696685] prod:(5.0, inf) ratio:(-inf, 1.2)
plane guess fit: [-0.2, -0.1, 1, -0.54, 0.2, -0.1, 1, -0.54]
use hexagons: False
enforce mirror symmetry: False
ff alpha: 0.1, max. iter.: 25
dFnl: [ 0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 -8.00453334e-02 -8.00453334e-02 -8.00453334e-02
-8.00453334e-02 -8.00453334e-02 -8.00453334e-02 7.62612083e-03
7.62612083e-03 7.62612083e-03 7.62612083e-03 7.62612083e-03
7.62612083e-03 3.68112793e-01 3.68112793e-01 3.68112793e-01
3.68112793e-01 3.68112793e-01 3.68112793e-01 4.65716488e-01
4.65716488e-01 4.65716488e-01 4.65716488e-01 4.65716488e-01
4.65716488e-01 8.17287237e-01 8.17287237e-01 8.17287237e-01
8.17287237e-01 8.17287237e-01 8.17287237e-01 5.92789810e-01
5.92789810e-01 5.92789810e-01 5.92789810e-01 5.92789810e-01
5.92789810e-01 3.85099098e-01 3.85099098e-01 3.85099098e-01
3.85099098e-01 3.85099098e-01 3.85099098e-01 2.43318538e-01
2.43318538e-01 2.43318538e-01 2.43318538e-01 2.43318538e-01
1.48217035e-01 1.48217035e-01 1.48217035e-01 1.48217035e-01
1.48217035e-01 1.48217035e-01 1.09508744e-01 1.09508744e-01
1.09508744e-01 1.09508744e-01 1.09508744e-01 1.09508744e-01
2.64204350e-02 2.64204350e-02 2.64204350e-02 2.64204350e-02
2.64204350e-02 2.64204350e-02 -1.26275695e-01 -1.26275695e-01
-1.26275695e-01 -1.26275695e-01 -1.26275695e-01 -1.26275695e-01
-1.39399786e-01 -1.39399786e-01 -1.39399786e-01 -1.39399786e-01
-1.39399786e-01 -1.39399786e-01 -1.22860948e-01 -1.22860948e-01
-1.22860948e-01 -1.22860948e-01 -1.22860948e-01 -1.22860948e-01
-1.66420706e-01 -1.66420706e-01 -1.66420706e-01 -1.66420706e-01
-1.66420706e-01 -2.26657858e-01 -2.26657858e-01 -2.26657858e-01
-2.26657858e-01 -2.26657858e-01 -2.26657858e-01 -3.43191302e-01
-3.43191302e-01 -3.43191302e-01 -3.43191302e-01 -3.43191302e-01
-3.43191302e-01 -4.03181635e-01 -4.03181635e-01 -4.03181635e-01
-4.03181635e-01 -4.03181635e-01 -4.03181635e-01 -4.54859594e-01
-4.54859594e-01 -4.54859594e-01 -4.54859594e-01 -4.54859594e-01
-4.54859594e-01 -3.63298806e-01 -3.63298806e-01 -3.63298806e-01
-3.63298806e-01 -3.63298806e-01 -3.63298806e-01 -3.57576383e-01
-3.57576383e-01 -3.57576383e-01 -3.57576383e-01 -3.57576383e-01
-3.57576383e-01 -2.65715514e-01 -2.65715514e-01 -2.65715514e-01
-2.65715514e-01 -2.65715514e-01 -1.66431840e-01 -1.66431840e-01
-1.66431840e-01 -1.66431840e-01 -1.66431840e-01 -1.66431840e-01
-8.14228426e-02 -8.14228426e-02 -8.14228426e-02 -8.14228426e-02
-8.14228426e-02 -8.14228426e-02 3.67432700e-02 3.67432700e-02
3.67432700e-02 3.67432700e-02 3.67432700e-02 3.67432700e-02
1.43658157e-01 1.43658157e-01 1.43658157e-01 1.43658157e-01
1.43658157e-01 1.43658157e-01 1.99958967e-01 1.99958967e-01
1.99958967e-01 1.99958967e-01 1.99958967e-01 1.99958967e-01
2.06103849e-01 2.06103849e-01 2.06103849e-01 2.06103849e-01
2.06103849e-01 2.06103849e-01 2.31494991e-01 2.31494991e-01
2.31494991e-01 2.31494991e-01 2.31494991e-01 2.31494991e-01
1.57034560e-01 1.57034560e-01 1.57034560e-01 1.57034560e-01
1.57034560e-01 1.34961857e-01 1.34961857e-01 1.34961857e-01
1.34961857e-01 1.34961857e-01 1.34961857e-01 1.07848008e-01
1.07848008e-01 1.07848008e-01 1.07848008e-01 1.07848008e-01
1.07848008e-01 6.15195621e-02 6.15195621e-02 6.15195621e-02
6.15195621e-02 6.15195621e-02 6.15195621e-02 4.21554264e-02
4.21554264e-02 4.21554264e-02 4.21554264e-02 4.21554264e-02
4.21554264e-02 2.18073533e-02 2.18073533e-02 2.18073533e-02
2.18073533e-02 2.18073533e-02 2.18073533e-02 1.46723419e-02
1.46723419e-02 1.46723419e-02 1.46723419e-02 1.46723419e-02
1.46723419e-02 7.35637424e-03 7.35637424e-03 7.35637424e-03
7.35637424e-03 7.35637424e-03 4.15214780e-03 4.15214780e-03
4.15214780e-03 4.15214780e-03 4.15214780e-03 4.15214780e-03
1.78038360e-03 1.78038360e-03 1.78038360e-03 1.78038360e-03
1.78038360e-03 1.78038360e-03 7.85856408e-04 7.85856408e-04
7.85856408e-04 7.85856408e-04 7.85856408e-04 7.85856408e-04
7.76728797e-05 7.76728797e-05 7.76728797e-05 7.76728797e-05
7.76728797e-05 7.76728797e-05 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 -7.22107260e-03 -7.22107260e-03
-7.22107260e-03 -7.22107260e-03 -7.22107260e-03 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00
0.00000000e+00 0.00000000e+00 0.00000000e+00 0.00000000e+00]
nl alpha:0.5, sat. level:500, nl max. iter.:25
[38]:
f = boz.inspect_correction(params, gain=params.gain)
mu: 0.5548827255333549, s: 0.005084289575244334, snr: 109.13672742699538
weighted mu: 0.5548458130246263, s: 0.0044434740849424535, snr: 124.86757037805747
diff mu: 0.5548827255333549, s: 0.004872386726144649, snr: 113.8831453086267
mu: 0.5976615112755194, s: 0.005382491809662051, snr: 111.03807166091065
weighted mu: 0.597788040850602, s: 0.0047850701948281725, snr: 124.92774745430208
diff mu: 0.5976615112755194, s: 0.005185181280175686, snr: 115.26337826616337
mu: 0.9285018768837483, s: 0.012200618602811707, snr: 76.10285241354643
weighted mu: 0.9281647927167087, s: 0.011232131843254469, snr: 82.63478435521786
diff mu: 0.9285018768837483, s: 0.011195132904929328, snr: 82.93799499914108
mu: 0.989131458619956, s: 0.0070755493535068804, snr: 139.79571185235378
weighted mu: 0.9884902174393602, s: 0.0054195731639837316, snr: 182.39263269079237
diff mu: 0.989131458619956, s: 0.007101403322843433, snr: 139.2867597645339
mu: 0.9920652557682886, s: 0.0066457310959286905, snr: 149.27857318452863
weighted mu: 0.9923455021819694, s: 0.005687673097417032, snr: 174.47302001808572
diff mu: 0.9920652557682886, s: 0.0066754073354702865, snr: 148.61493927072797
mu: 0.9970452858694611, s: 0.0033459909372665136, snr: 297.982064076955
weighted mu: 0.9961149773600704, s: 0.0029137435593010515, snr: 341.86775777859407
diff mu: 0.9970452858694611, s: 0.003264412741680508, snr: 305.4286834317972
mu: 0.9969613645782567, s: 0.002526641087343409, snr: 394.5797325834251
weighted mu: 0.9967346659245049, s: 0.0021407377892240045, snr: 465.60334055942974
diff mu: 0.9969613645782567, s: 0.002537198385132514, snr: 392.9378839353892
mu: 0.9990953203422078, s: 0.002431422225116063, snr: 410.9098411710522
weighted mu: 0.9993800049741802, s: 0.0020677924999526415, snr: 483.3076844011519
diff mu: 0.9990953203422078, s: 0.002429860501200696, snr: 411.1739418162125
mu: 0.9978674182119845, s: 0.0026633668482404335, snr: 374.6639028984049
weighted mu: 0.9973530198357894, s: 0.0023843840153367555, snr: 418.2853992564321
diff mu: 0.9978674182119845, s: 0.0025864547554682957, snr: 385.8050932854279
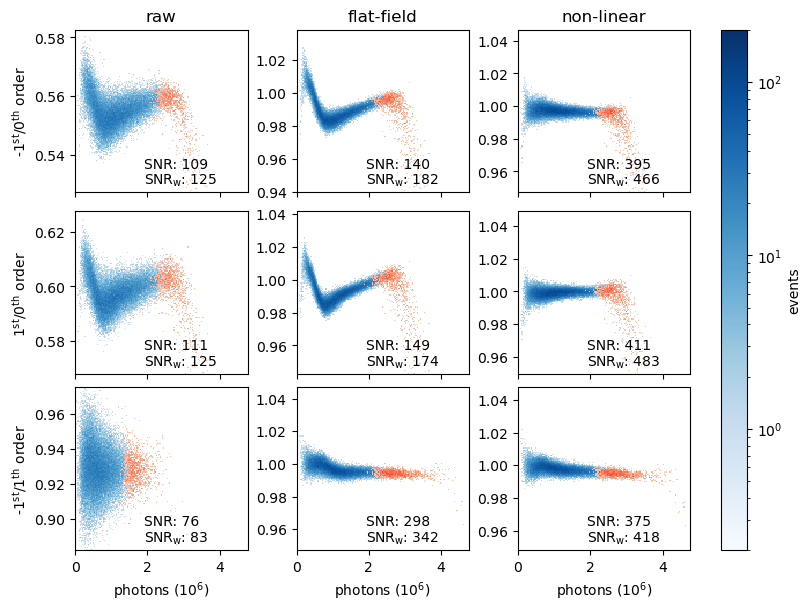
[39]:
f.savefig(path+prefix+'-inspect-correction.png', dpi=300)
plotting the fitted correction¶
[40]:
f = boz.inspect_Fnl(boz.ensure_on_host(params.get_Fnl()))
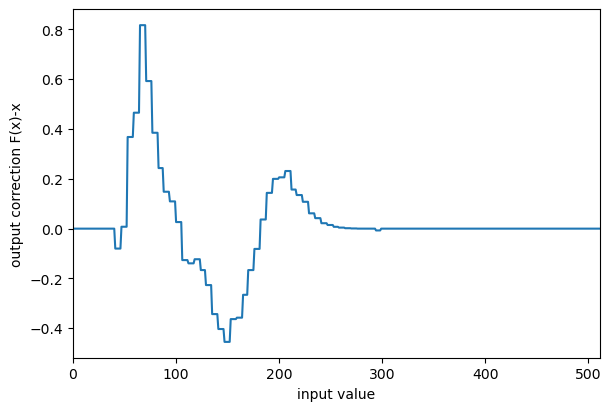
[41]:
f.savefig(path+prefix+'-inspect-Fnl.png', dpi=300)
plotting the fit progresion¶
[42]:
f = boz.inspect_nl_fit(fit_res)
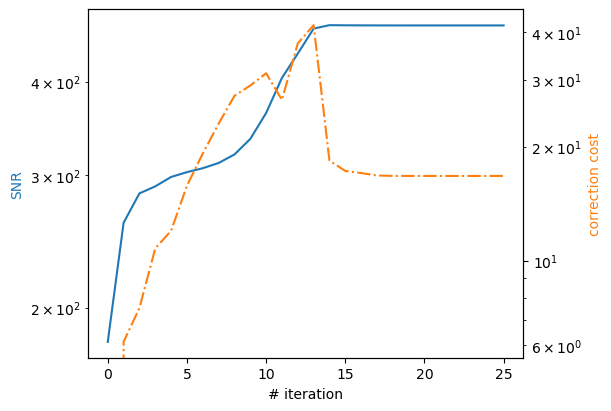
[43]:
f.savefig(path+prefix+'-inspect-nl-fit.png', dpi=300)
Save the analysis parameters¶
[44]:
params.save(path=path)
/gpfs/exfel/exp/SCS/202122/p002937/usr/processed_runs/r0614/parameters_p2937_d615_r614.json